iOS Keyboard SDK
iOS Keyboard SDK Documentation
Revisions:
Version | Details | Date | Author |
---|---|---|---|
1.0 | Initial Version | 11/06/2021 | MT |
1.1 | Note added for activating font for selected keyboard | 20/07/2021 | MT |
1.4.0 | Update includes:
| 20/01/2022 | VW |
1.4.1 | Update includes:
| 01/03/2022 | VW |
1.5.1 | Update includes:
| 22/03/2022 | VW |
1.6.0 | Update includes:
| 29/04/2022 | VW |
1.7.1 | Update includes:
| 20/05/2022 | VW |
2.0.6 | Update includes:
| 01/08/2022 | VW |
2.0.7 | Update includes:
| 26/08/2022 | VW |
2.0.10 | Update includes:
| 29/09/2022 | VW |
Prerequisites
• Your application should be set up to use Cocoapods https://cocoapods.org
• You will require an active Apple developer account with signing capabilities
Getting Started
Follow these instructions to add the Kindred SDK to an existing iOS application.
For any questions or support with your integration, or to receive your API details, please email [email protected]
-
We need to add a keyboard target to your app. Within Xcode, select File -> New -> Target and add a Custom Keyboard Extension and give your Extension a name.
-
Add the KindredSDK to your podfile.
source 'https://github.com/kindred-app/Specs.git'
target 'your_app_name' do
pod 'KindredSDK', '2.0.10'
end
target 'your_keyboard_target' do
pod 'KindredSDK', '2.0.10'
end
-
In terminal, navigate to your project folder and run: -
pod install -
You will now need to set up app groups within your Apple developer account.
To create a new App Group, do the following:
- Visit Apple's iOS Developer Centre, open your Account and log in.
- Select Certificates, IDs & Profiles.
- Under Identifiers select App Groups and click the + button to create a new group.
- Enter a Name and an Identifier for the new group and click the Continue button:
- Click the Register button to create the group and the Done to return to the list of registered App Groups.
- Now, back in Xcode, go to the Project Navigator -> Select your applications target -> Head to the Signing and Capabilities tab -> Click the + button and search for groups. Add an App Group.
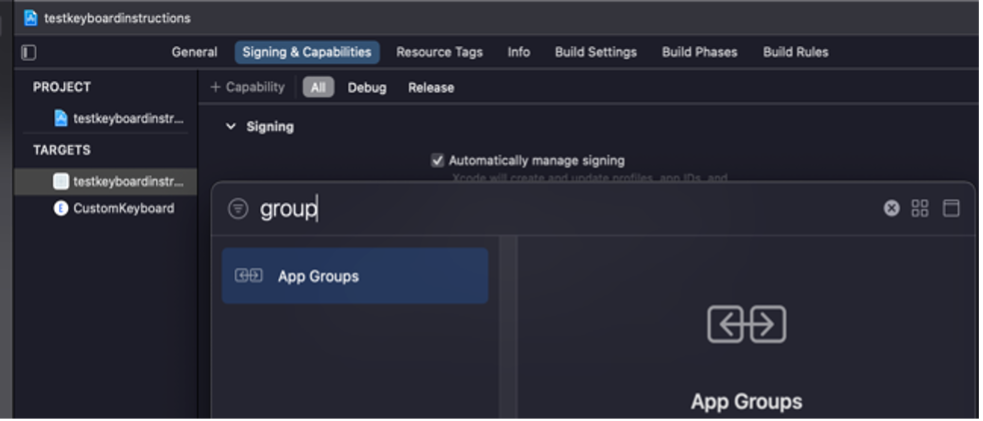
Important Note: Repeat this step for your Keyboard Extension target.
-
Head to your application info.plist and add a key for AppGroupName with a string value matching the group name you created in step 4.
-
Now head to the keyboard extension info.plist and add a key for AppGroupName with the same string value as steps 4, 5 and 6.
-
Still within your keyboard extension info.plist file, now add a dictionary key named KindredKeyboard with 5 string values named, AuthClientId, AuthClientSecret, AuthSharedKey, BaseApiUrl, CDNUrl. This should look like the following: -
Note: Contact[email protected] to acquire your credentials.
<key>KindredKeyboard</key>
<dict>
<key>AuthClientId</key>
<string>YOUR_AUTH_CLIENT_ID</string>
<key>AuthClientSecret</key>
<string>YOUR_CLIENT_SECRET</string>
<key>AuthSharedKey</key>
<string>YOUR_SHARED_KEY </string>
<key>BaseApiUrl</key>
<string>https://api-partners.kindred.co</string>
<key>CDNUrl</key>
<string>https://cdn.kindred.co</string>
</dict>
Note: Still within the keyboard extension info.plist, expand NSExtensionAttributes and make sure RequestsOpenAccess value is set to 1.
Note: This is important to grant keyboard access.
- Within the extension KeyboardViewController , replace all existing code with the following: -
import UIKit
import KindredSDK
class KeyboardViewController: KindredViewController {
convenience init() {
self.init(nibName:nil, bundle:nil)
self.setUserId(userId: "YOUR_USER_ID")
}
override func configureKeyboard() -> CustomerKeyboardConfiguration {
return CustomerKeyboardConfiguration(
display: KeyboardDisplayConfiguration(
cashbackLabel: KeyboardDisplayConfiguration.cashbackLabelType(
type: KeyboardDisplayConfiguration.CashbackLabel.CASHBACK),
showWithdrawal: true
),
user: UserConfiguration(userCountry: "UK", userCurrency: "GBP")
)
}
}
Note: The setUserId function simply sets a value in UserDefaults which can be used later, you can also set this value in your application by saving the value to UserDefaults using the key KKUserId. Example below: -
let defaults = UserDefaults.standard
defaults.setValue("YOUR_USER_ID", forKey: "KKUserId")
Note: replace YOUR_USER_ID with your user ID value
-
At this point we have set up the Kindred keyboard however, to add the logo we need to create an xcassets folder within your keyboard extension.
-
Select the keyboard extension within Xcode -> Right Click -> New file -> Asset Catalogue, name the folder images.
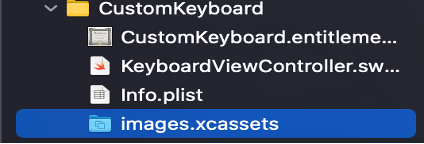
- Within the images.xcassets folder we need to add 2 image assets named LogoActive and LogoInactive. These should both contain images assets for 1x, 2x & 3x respectively. E.g. -
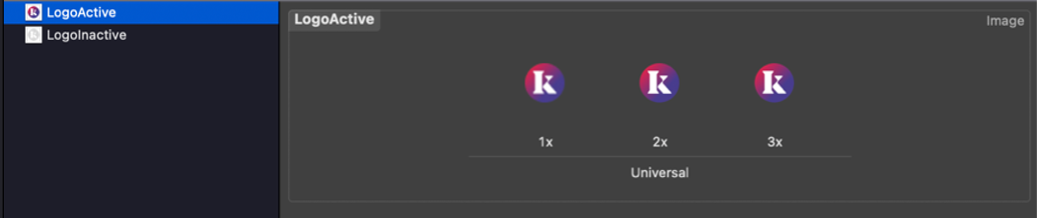
-
We now need to add fonts to the keyboard target. Right click your keyboard target -> New group -> Call the folder fonts
-
Download the font from flicon.ttf
-
Drag the flicon.ttf font into the newly created font folder and make sure to check “copy items if needed” then click Finish.
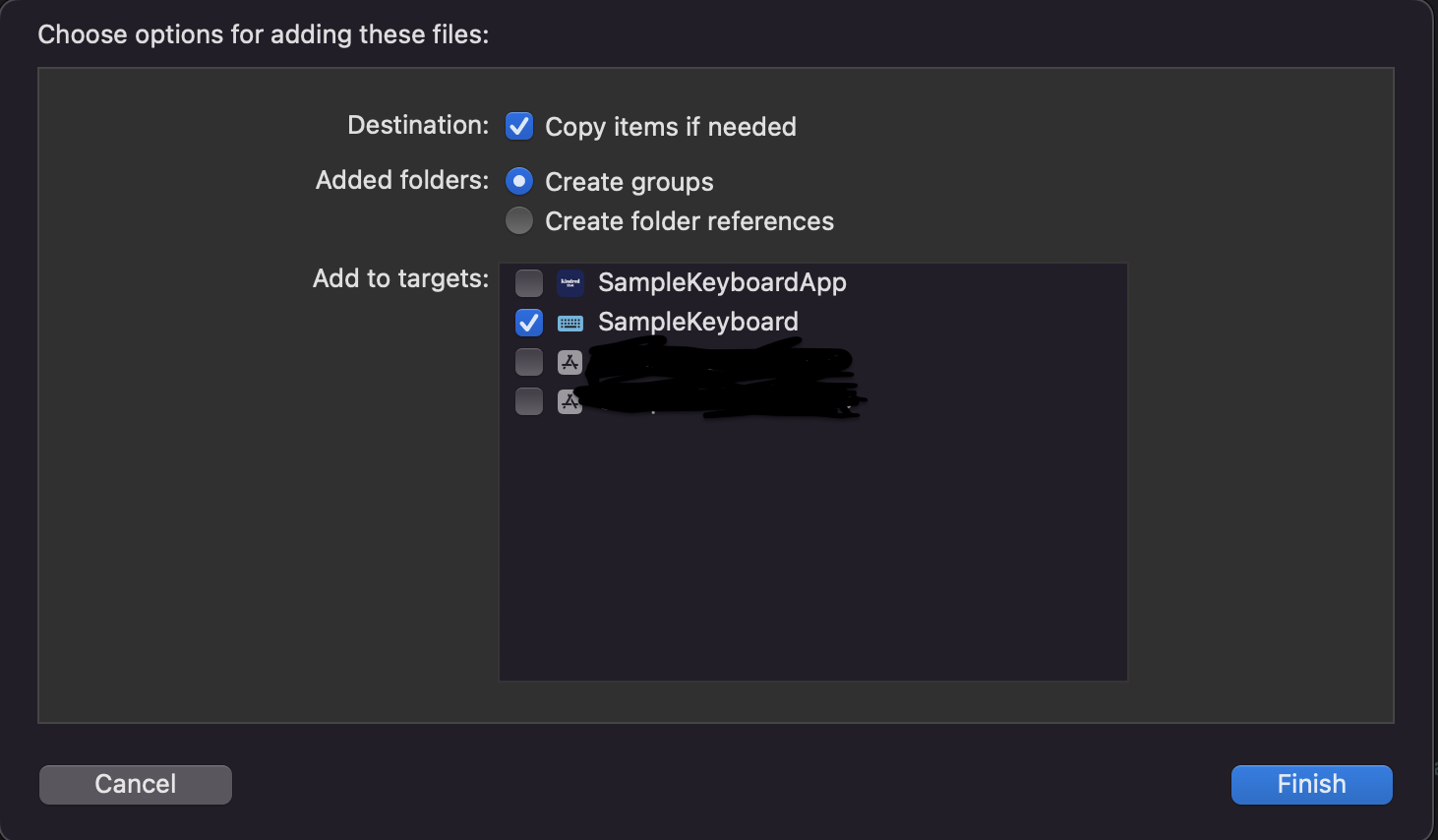
NOTE: Within Xcode file explorer, select the font you just added then on the right hand column in Xcode make sure to select your keyboard target for the font (see below)

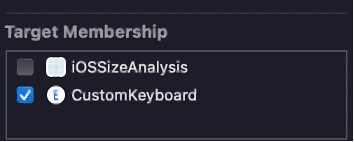
- Inside your keyboard target info.plist add a key for “Fonts provided by application” with a value of “flicon.ttf” It should look like the following:

-
If you now close Xcode and head to your application project folder and open the xcworkspace you should now be able to run the keyboard project.
-
Remember to enable the keyboard and allow permissions in the iOS settings app.
Settings -> General -> Keyboard -> Keyboards -> Add New Keyboard
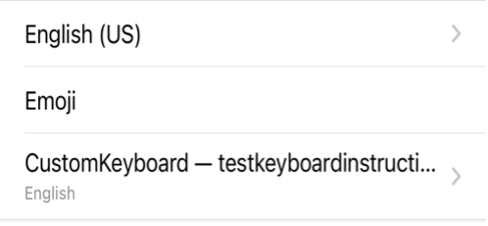
Settings -> General -> Keyboard -> Keyboards -> YourKeyboard -> Allow Full Access
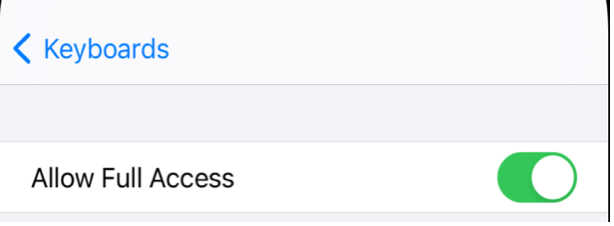
Note: To test deals you will need to use a browser.
Keyboard Settings Bundle
Summary
Name | Description |
---|---|
Auto-Correction | Enable / Disable Auto-Correction |
Auto-Capitalization | Enable / Disable Auto-Capitalization |
Double-Tap-Period | Enable / Disable two taps add a period |
Delete-Correction | Enable / Disable Reverse Correct |
Show-Word-Predictions | Enable / Disable the top bar of predictions |
Autolearn-From-User | Enable / Disable the ability to learn words |
Emoji-Suggestion | Enable / Disable Suggest Emoji to Replace Words |
Emoji-Prediction | Enable / Disable Emoji Prediction |
Swipe-Typing | Enable / Disable Swipe Typing |
Report-Analytics | Enable / Disable report of internal analytics |
Speak | Enable / Disable voice feedback for the user |
Swipe-Length | Threshold before trigger swipe gesture |
Underline-Spell-Check | Enable / Disable allow underline |
Typing-Sounds | Enable / Disable Typing Sounds |
All Settings Reference
Full Name | Type | Default Value | Description |
---|---|---|---|
com.kindred.setting.userAutoCorrection | Boolean | true | Enable/Disable auto correction. Automatically auto correct written text. |
com.kindred.setting.userAutoCapitalization | Boolean | true | Enable/Disable auto capitalisation. Automatically capitalise letters when it’s at the beginning of the sentence, etc. |
com.kindred.setting.doubleTapPeriods | Boolean | true | Enable/Disable two taps add a period |
com.kindred.settings.deleteCorrection | Boolean | false | Enable/Disable reverse-correct when you press delete after one correction. |
com.kindred.setting.showWordPredictions | Boolean | true | Enable/Disable the top bar of predictions. If enabled it shows the predictions in the top bar, otherwise no. |
com.kindred.settings.autolearnFromUser | Boolean | true | Enable/Disable the ability to learn words from the user while it is typing, automatically. |
com.kindred.settings.emojiSuggestion | Boolean | true | Enable/Disable emoji suggestion. Emoji suggestion triggers when we recognise a word that could be exchanged by an emoji |
com.kindred.settings.emojiPrediction | Boolean | true | Enable/Disable emoji prediction. After space, we look for possible new emojis after the current word. |
com.kindred.settings.swipetyping | Boolean | true | Enable/Disable swipe typing for the keyboard. |
com.kindred.settings.reportAnalytics | Boolean | false | Enable/Disable report of internal analytics which includes autocorrections, ... Check reportAnalytics method to get more of it. |
com.kindred.setting.speak | Boolean | false | Enable/Disable voice feedback for the user.
|
com.kindred.settings.swipeLength | Integer | 60 | This setting adjusts the threshold before trigger swipe gesture. By default, this is 60. Do not modify unless you know exactly what you are trying to achieve |
com.kindred.settings.underlineSpellCheck | Boolean | false | Enable / Disable to allow underline colour in textfield on spell check |
com.kindred.settings.typingSounds | Boolean | true | Enable / Disable typing sounds |
Setup
To add a Settings Bundle to your application you will need to action the following steps: -
- Within Xcode Project navigator, select your application -> Right Click -> New File -> Select Settings Bundle. Leave the default name of “Settings”
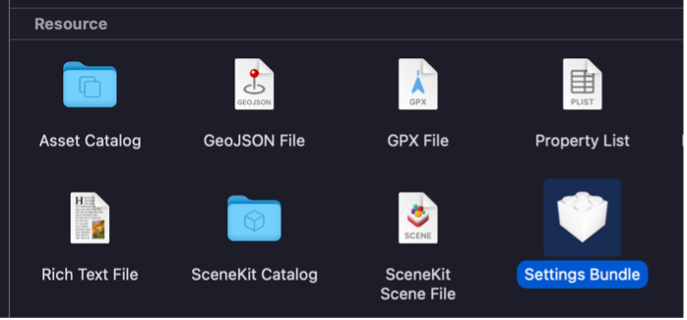
-
Once you have added the settings bundle to your project you will see a new bundle containing a en.Iproj folder and a root.plist file.
-
Right click the root.plist file and select Open As -> Source Code
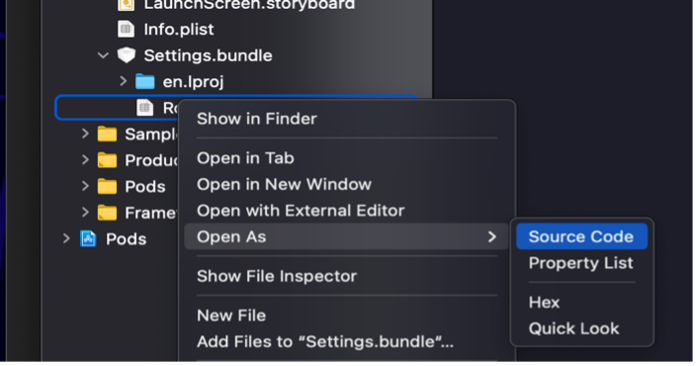
- Replace the existing contents of the file with the following xml: -
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
<key>ApplicationGroupContainerIdentifier</key>
<string>group.com.your_group_string</string>
<key>StringsTable</key>
<string>Root</string>
<key>PreferenceSpecifiers</key>
<array>
<dict>
<key>Type</key>
<string>PSGroupSpecifier</string>
<key>FooterText</key>
<string>There's only 3 steps to start using the Keyboard
1. Select "Keyboards"
2. Turn on "Kindred Keyboard"
3. Turn on "Allow Full Access"
*If "Keyboards" does not appear on this screen:
- If you have an iPhone 8 or earlier:
Please double-click the Home button, swipe up the
"Settings" and reopen "Settings" again. Then repeat the
operations above.
- If you have an iPhone X or later:
Please swipe up from the bottom to the middle of your
screen and swipe up the "Settings". Reopen "Settings"
again and then repeat the operations above.</string>
</dict>
<dict>
<key>Type</key>
<string>PSGroupSpecifier</string>
<key>Title</key>
<string>Keyboard Settings</string>
</dict>
<dict>
<key>TrueValue</key>
<true/>
<key>FalseValue</key>
<false/>
<key>DefaultValue</key>
<true/>
<key>Type</key>
<string>PSToggleSwitchSpecifier</string>
<key>Title</key>
<string>Auto Correction</string>
<key>Key</key>
<string>com.kindred.setting.userAutoCorrection</string>
</dict>
<dict>
<key>DefaultValue</key>
<true/>
<key>Type</key>
<string>PSToggleSwitchSpecifier</string>
<key>Title</key>
<string>Auto Capitalization</string>
<key>Key</key>
<string>com.kindred.setting.userAutoCapitalization</string>
</dict>
<dict>
<key>DefaultValue</key>
<true/>
<key>Type</key>
<string>PSToggleSwitchSpecifier</string>
<key>Title</key>
<string>Double Tap Period</string>
<key>Key</key>
<string>com.kindred.setting.doubleTapPeriods</string>
</dict>
<dict>
<key>DefaultValue</key>
<true/>
<key>Type</key>
<string>PSToggleSwitchSpecifier</string>
<key>Title</key>
<string>Show Word Predictions</string>
<key>Key</key>
<string>com.kindred.setting.showWordPredictions</string>
</dict>
<dict>
<key>DefaultValue</key>
<true/>
<key>Type</key>
<string>PSToggleSwitchSpecifier</string>
<key>Title</key>
<string>Autolearn From User</string>
<key>Key</key>
<string>com.kindred.settings.autolearnFromUser</string>
</dict>
<dict>
<key>DefaultValue</key>
<true/>
<key>Type</key>
<string>PSToggleSwitchSpecifier</string>
<key>Title</key>
<string>Emoji Suggestion</string>
<key>Key</key>
<string>com.kindred.settings.emojiSuggestion</string>
</dict>
<dict>
<key>DefaultValue</key>
<true/>
<key>Type</key>
<string>PSToggleSwitchSpecifier</string>
<key>Title</key>
<string>Emoji Prediction</string>
<key>Key</key>
<string>com.kindred.settings.emojiPrediction</string>
</dict>
<dict>
<key>DefaultValue</key>
<true/>
<key>Type</key>
<string>PSToggleSwitchSpecifier</string>
<key>Title</key>
<string>Typing Sounds</string>
<key>Key</key>
<string>com.kindred.settings.typingSounds</string>
</dict>
<dict>
<key>DefaultValue</key>
<true/>
<key>Type</key>
<string>PSToggleSwitchSpecifier</string>
<key>Title</key>
<string>Swipe Typing</string>
<key>Key</key>
<string>com.kindred.settings.swipetyping</string>
</dict>
<dict>
<key>DefaultValue</key>
<false/>
<key>Type</key>
<string>PSToggleSwitchSpecifier</string>
<key>Title</key>
<string>Underline Spell Check</string>
<key>Key</key>
<string>com.kindred.settings.underlineSpellCheck</string>
</dict>
</array>
</dict>
</plist>
Note: Make sure to change the ApplicationGroupContainerIdentifier (line 6) to match your App group identifier that you setup in step 4 of this setup guide
API Reference
The Kindred SDK gives several different settings that can be configured based on the commercial agreement with Kindred. These settings will define whether your users receive cashback and donate some of this to charity OR all of the savings are recognised as a donation, whether payout of any user savings is via Kindred or via yourselves, and settings that define location and currency for each user. Below are the key settings and the different options that should be selected based on your agreement.
Summary
CashbackLabelType | Enum value for cashback type |
configureKeyboard | Invoked when the keyboard requires a |
KeyboardDisplayConfiguration | Optional class. Keyboard display settings |
AuthConfiguration | Optional class. Authentication settings |
UserConfiguration | Required class. User configuration settings |
SettingsConfiguration | Internal class. Keyboard Settings Configuration |
LogoActive, LogoInactive | Icon to be displayed at top-left of keyboard |
setUserId | Saves UserID to UserDefaults |
showWithdrawal | Boolean value to set withdrawal button visible |
userCountry | String value to set deals for country |
Functions
configureKeyboard
configureKeyboard |
---|
This function is called upon init and is responsible for the keyboard configuration, override this method and provide KeyboardDisplayConfiguration, AuthConfiguration, UserConfiguration etc. |
setUserId
The UserId denoted below is used to uniquely identify users on your platform, and on the Kindred platform. It is the ID that will be used to link the user data from both sides. If your platform allows a single user account to be used on multiple devices, such as an iPad and iPhone or is device agnostic, you should ensure that the UserId is specific to the user and not the device.
setUserId |
---|
This function saves userId String value to UserDefaults. You may also save the value yourself to UserDefaults using the key KKUserId. |
Classes
KeyboardDisplayConfiguration
There are two key settings which will define the users’ keyboard experience;
- Whether users will be able to withdraw any cashback through a Kindred hosted and co-branded wallet OR pay-out to users of their cashback will be done via your app/services or converting those savings into in app credit, points or in game currency.
- Whether users will be earning cashback which they can choose to donate a percentage of, OR if all their cashback is converted into a donation.
showWithdrawal is used to define whether users will see a button on the keyboard prompting them to create a Kindred account to allow them to withdraw cashback via Kindred in a co-branded wallet area. If the button is shown, then users will be prompted to create a Kindred account. Thereafter, they will be able to view their earned cashback, available balance, and withdraw funds once the threshold has been met.
CashbackLabelType is used to set whether or not a user sees that they will be earning cashback and choosing to donate some of their savings, or donating all of their savings to charity through the UI.
The key UI experiences are:
Donations
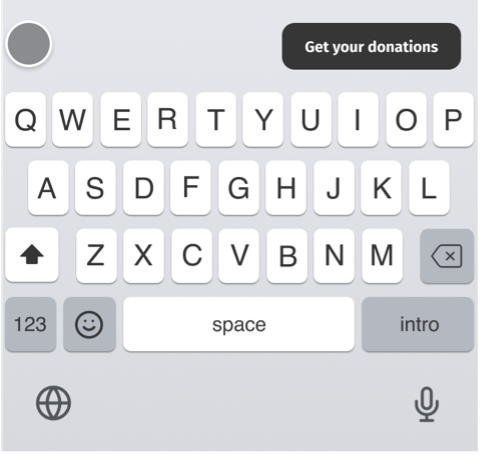
Pre-Sign Up Button
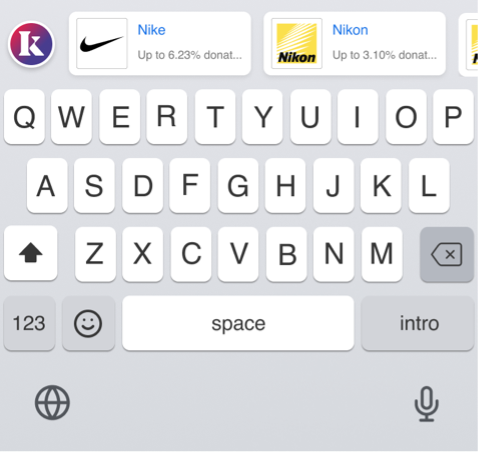
Deal Tiles with Donation
Cashback
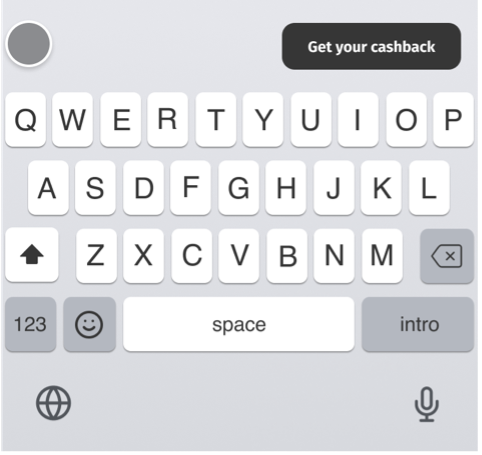
Pre-Sign Up Button
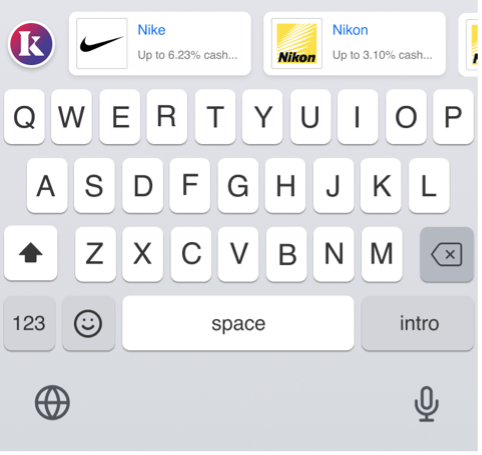
Deal Tiles with Cashback
KeyboardDisplayConfiguration - Optional |
---|
This class is responsible for the display configuration of the Keyboard. The class accepts two optional properties cashbackLabel of type CashbackLabelType and showWithdrawal of type Bool. |
|
|
SettingsConfiguration
SettingsConfiguration |
---|
This class is responsible for all keyboard related settings. Call SettingsConfiguration.shared.saveSettings() in KeyboardViewController's init. |
UserConfiguration
This defines the setup for each user as it relates to their location and currency.
Location will be used to filter the deals shown to each user, restricting the deals to the specified shipping country.
Currency will be used for all users and all cashback and/or donations received will be converted to this base currency and either paid directly to you or to the user depending on your agreement with Kindred.
UserConfiguration – Required |
---|
This class is responsible for any user related settings. A UserConfiguration must be supplied to CustomerKeyboardConfiguration |
|
|
CharityConfiguration
This defines which charity will receive the donations from each users’ earnings as well as the percentage split that will be made. These settings are independent of the display settings above.
CharityId is a valid Charity Id either received from the Kindred API or sent directly by the Kindred SDK Support Team based on your agreement. This is set per user.
CharityShare is the percentage of cashback that is donated to the selected charity from every received transaction for that user.
CharityConfiguration |
---|
This class is responsible for all charity related settings. Provide CharityConfiguration to CustomerKeyboardConfiguration class in overridden configureKeyboard function. CharityConfiguration expects charityId of type String and charityShare of type Int. |
| ID String of charity to receive donations. |
| Int value of charity share amount. E.g., 5 = 5% |
KindredInterface
This class contains the following static functions:
public static func isKeyboardExtensionEnabled() -> Bool
This function is used to determine whether the keyboard extension is enabled in the settings.
public static func hasFullAccess(appGroupName: String?) -> String
This function is used to determine whether full access setting is enabled for the keyboard extension. It takes the string representing the AppGroupName which is set in the info.plist as a parameter and returns a string from unknown/true/false
public static func isKeyboardActive(textField: UITextField?) -> String
This function is used to determine whether the custom keyboard is current active keyboard. It takes an UITextField object as a parameter and returns a string from unknown/true/false
public static func openSettings(app: UIApplication)
This function open the Settings app. It takes an UIApplication object as a parameter. Pass UIApplication.shared as the parameter.
KindredInterface.openSettings(app: UIApplication.shared)
Other
The file should be 40x40 and either a jpg or png
LogoActive - Logo displayed at the top left of the keyboard. | LogoInactive - Logo displayed at the top left of the keyboard. |
If keyboard full access is granted LogoActive is displayed | If keyboard full access is not granted LogoInactive is displayed. |
Troubleshooting
- If you get the following error after pod install :

update the podfile to
source 'https://github.com/kindred-app/Specs.git'
target 'your_app_name' do
pod 'KindredSDK', '2.0.10'
target 'your_keyboard_target' do
pod 'KindredSDK', '2.0.10'
end
end
and run pod install
- If you get the following error after pod install

or your pod version in Podfile.lock is not the same as podfile, run pod install --repo-update
- Missing Info.plist
If there is no Info.plist in your project navigator, you can make the changes to the Info tab of the target settings
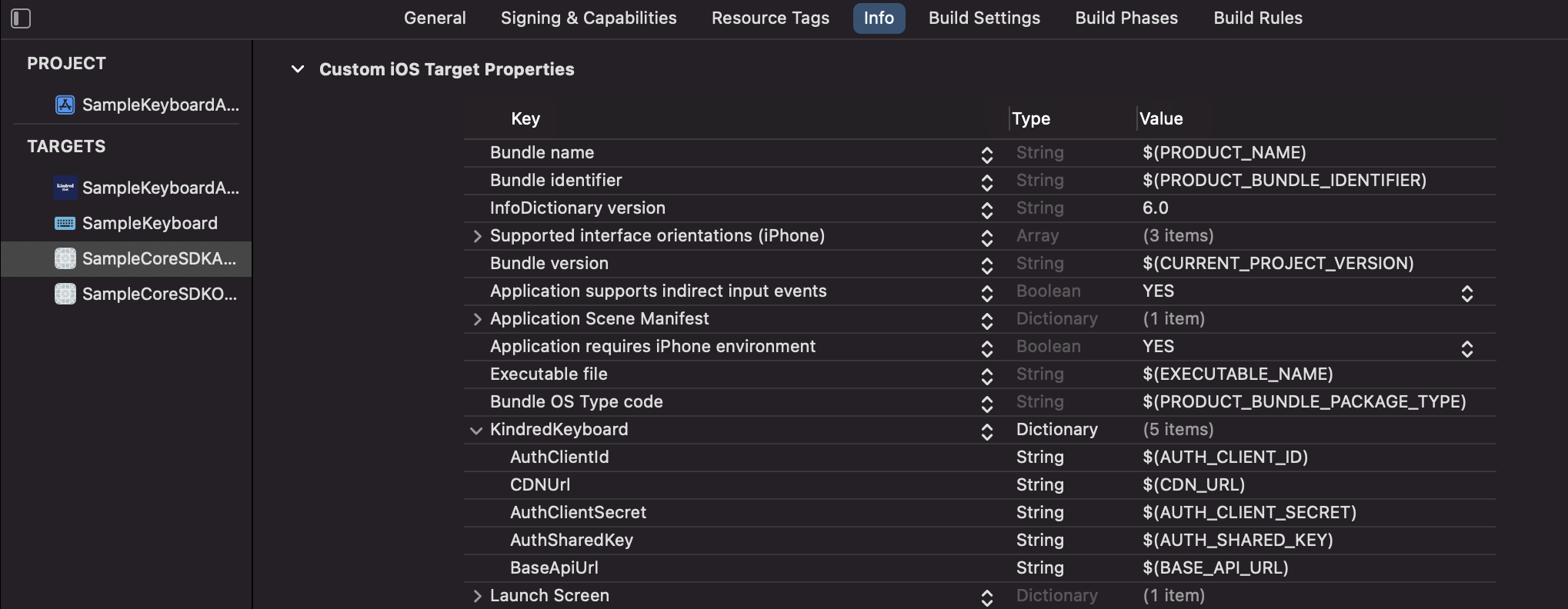
Updated 13 days ago